Finished 7 Billion Humans (PC) + all size+speed optimizations, all achievements
This is a programming-themed game, similar to Human Resource Machine, where you have a drag-and-drop interface to program in a pseudo-assembly type thing.
The big difference is 7 Billion Humans is "multithreaded". You program the actions of not just one worker, but many (sometimes, dozens) that all run the same program concurrently. There are some instructions "tell" and "listen" which help synchronize. For example one worker can tell another "Coffee time!", unblocking another worker that has stopped, and is listening for Coffee Time. Workers can be in eachothers' way, and you may want to avoid them taking paths where they collide with each other. Certain workers have resources dedicated to them, that others cannot use or else BOOM they explode (literally).
Compared to HRM and most computer assembly languages, 7BH has some conspicuously powerful instructions.
When optimizing for speed you come to learn about how the game computes "performance cost" since it is not totally intuitive.
For example,
if (X == 5 and Y == 3) or Z == 12342
is the exact same cost as
if (X == 5)
Also,
"Take two steps to the left"
can (in, at least some circumstances) be the exact same cost as
"Find out where in the room the nearest printer is- it might be around a maze of hazards, obstacles, whatever- and go to it"
The latter, you'd think would be an expensive and complicated path-finding operation but the game gives that one to you for free. Maybe, with the idea that you've got enough other things to deal with and it is probably right about that.
My solutions are in this GitHub repo
https://github.com/clandrew/7bh
"Things you need a PhD in math to do" (according to a programmer?)
- Does the graph of the equation "abs(x) + abs(y) = 2" look like a circle or a square?
- Use combinatorics to answer this: you have a collection of six things and need to choose two of them. How many different ways are there to do that?
- Figure out how old your child is when you give a quantity in months and your child is older than 1 year old?
- What month is six months before November?
- Know that adding up some numbers, then dividing that sum by how many numbers there were, gives you an average
- If you know two side lengths of a triangle and the angle between them, find the length of the third side.
- (uncommon but still a thing) Your child is older than two and you tell me how old they are in a quantity of months
Sometimes I rely on our resident mathematics expert and his expertise in this area, in cases where the rest of us can't put our heads together and solve it. In other cases, I've managed to hammer these answers out myself. But don't worry, my Fields medal acceptance speech is already underway, at least I've gotten the main points written out and the main parties I should thank.
Got all size+speed optimization challenges in Human Resource Machine!
Some of them are HARD.
I have new appreciation for being able to std::swap (in one expression), or use, like, any literals
And the ending. The reward is a creepy cutscene...
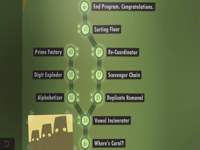
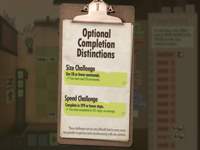
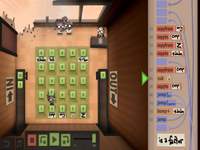
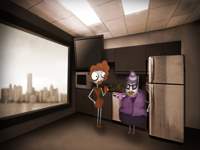
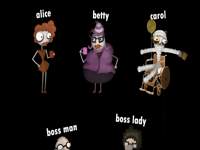
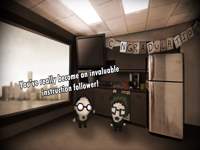
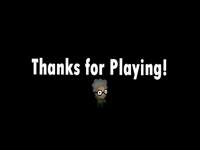
I would agree with Mr Stark but then we would both be wrong.
This game was a team effort with myself and 4 other students, for a contest called Games4Girls. The contest is run by University of Illinois. The objective is to make a 2D game, of any kind, targeted toward a female highschool-age demographic, with the restriction that we use Game Maker. Game Maker is a proprietary program used for scripting 2D games, using a C-like language. The choice of Game Maker had something to do with problems with past entries that depended on a wide assortment of platforms/runtimes. Many games wouldn't run. So, they ruled that all entries had to use Game Maker.
We decided to make a platformer type of game, which is familiar to most people. The player controls a character named Alessa, and uses her various weapons to defeat enemies and move through the levels.
Because there were restrictions on using copyrighted content, we created all the graphics and music ourselves.
My personal role was
- coding object collisions
- coding scene transitions, scoring
- creating sprite and background graphics and getting them into the game
Overall the project was a lot of fun to work on. As it turned out, our entry was very well-recieved and we finished in first place. We won a cash prize for our team and some money donated to our CS faculty at University of Waterloo.
https://info.uwaterloo.ca/www/profiles/research_profiles/profile-archive.php?id=193
The levels contain various enemies and obstacles.
The game includes some dialogue segments, illustrated by our group, with larger character graphics illustrated by my super-artistically-inclined groupmate! The dialogue provides some story background to the game.
Controls:
- Left/right arrows: move Alessa
- Up arrow: Jump
- Any arrow(in water): swim
- A key: Shoot an arrow
- S key: Swing a sword
My team consisted of (big thanks to my teammates!):
The game was originally built and tested on a Windows 7 environment.
Notes for running on Windows 10 and later:
- Windows may mark the game executable as protected by SmartScreen. Although anything downloaded here is at your own risk, I attest that we did not put malware into the game and do not distribute it knowingly with any malware. To proceed past the SmartScreen filter should you choose to do so click "More Info" and "Run".
- The Game Maker environment relies on DirectPlay which, from Windows 7 to 10, was changed from a built-in feature of the OS into an optional component which is downloaded on demand. If Windows prompts you to download it, choose Yes to proceed with downloading it and enable playing the game.
This is a game of Tetris written in x86 assembly, in a personal effort to learn more about the instruction set. It uses mode 13h for all drawing, writing directly to address 0xA000 to plot. The index written for each pixel determines the color in a 256-indexed color palette.
It uses DOS interrupts for other functionality like random number generation, and keyboard interaction. For random numbers, it uses the modulus of the current system time. The game keeps going until pieces reach the top of the screen.
The keys i,j,k, and l (or arrow keys for the Win32 version) are used to move the pieces.
In the normal way, rows are cleared when pieces occupy the entire row with no holes.
The form of each 'tetrad' (piece) is hardcoded at the top of the source file, and used for drawing the grid and checking for collisions. The walls of the game are fixed. An array called 'predict' is used to foreshadow where the user is trying to move the tetrad, so that it cannot be moved through a wall or other landed pieces.
After every 'tick' of the game, the current tetrad in play is moved down by one square if possible, or committed to the grid if there are other pieces blocking the way. The game determines the timing using an interrupt, and advances the game after 10 time units, which is around every half a second.
Download binary (DOS com executable)
The source code was assembled using the A86 assembler.
-----------------------------------
Update (5/14/2018): Since the time of writing and building this program, the binary has rotted 🙁
However, I ported the code to Win32 with GDI and built it as a 32-bit PE executable.
Here's the newly updated Windows version:
Download binary (32-bit Windows executable)
The Win32 source code was assembled using MASM.
View Source File or Download Visual Studio 2018 Project (zip)
The Windows version also has the following changes:
- Different colors for the different pieces
- Fixed a bug where rows would sometimes not get cleared correctly
- Added a 'next piece' UI
- When you get game over instead of crashing it displays a message and you can press Escape to start again
Besides i,j,k,l you can also use the arrow keys.